Using the Blazor programming model to handle button events, like OnClick, makes writing Xamarin mobile applications with form inputs a breeze.
Getting Started
In the previous tutorial, you learned how to create some of the more common mobile app layout designs. In this tutorial, we will bind the values of some Entry
components and do something with them when a button is clicked.
If you are used to web development, the Entry
component is basically the mobile equivalent of an input
field, or an InputText
component in traditional Blazor web applications.
Designing the Form
For demonstrations purposes, the form itself will be quite simple. In fact, we will create a mobile equivalent of a C# console application used in the C# for Beginners tutorial series. It will ask for a user's first name, last name, and the year of their birth. Finally, it will perform some simple calculations and return some results to display in the the mobile app user interface.
For the form itself, I will use a simple StackLayout with Label and Entry components to collect the user's input.
<StackLayout Margin="new Thickness(16)">
<Label Text="Enter your first name?"
FontSize="16" />
<Entry @bind-Text="firstName" />
<Label Text="Enter your last name?"
FontSize="16" />
<Entry @bind-Text="lastName" />
<Label Text="What year were you born?"
FontSize="16" />
<Entry @bind-Text="birthYearString" />
<Button OnClick="ButtonClickHandler" Text="Submit" />
</StackLayout>
@code {
string firstName;
string lastName;
string birthYearString;
private void ButtonClickHandler()
{
}
}
A note about the Xamarin Entry
component is that it binds to a string
. In order to bind to an int
, you can use a ValueConverter. In this example, I will just perform the conversion myself in the ButtonClickHandler()
method, and I will display an error message if a non-integer value is entered.
string errorMessage;
private void ButtonClickHandler()
{
if (int.TryParse(birthYearString, out int birthYear))
{
errorMessage = null;
//Do something with birthYear int
}
else
{
errorMessage = "Please enter a valid year.";
}
}
So far, my button's OnClick handler will try to parse the string entered into the birthYearString
Entry field into an integer value. If it succeeds, we can do something with the result. If it fails, we can display an error message for the user. Let's show the error message on the screen above the Submit button.
@if (errorMessage != null)
{
<Label Text="@errorMessage" TextColor="Color.Red"
FontSize="14" />
}
<Button OnClick="ButtonClickHandler" Text="Submit" />
Displaying the Results
To hold the results, I will create a model that contains the three strings we want to display to the user. Create a new class file called ResultModel.cs.
public class ResultModel
{
public string WelcomeText { get; set; }
public string CurrentAgeText { get; set; }
public string PlusFiveAgeText { get; set; }
}
Then, in your button's OnClick handler, you can perform any necessary calculations and store the results in a ResultsMode
l object.
ResultModel result;
private void ButtonClickHandler()
{
if (int.TryParse(birthYearString, out int birthYear))
{
errorMessage = null;
int currentAge = DateTime.Now.Year - birthYear;
result = new ResultModel()
{
WelcomeText = $"Hello, {firstName} {lastName}.",
CurrentAgeText = $"This year, you will be {currentAge} years old.",
PlusFiveAgeText = $"In 5 years, you will have {currentAge + 5} years."
};
}
else
{
result = null;
errorMessage = "Please enter a valid year.";
}
}
Finally, display the results to the user. You could pass the ResultModel
object as a parameter to a separate Razor component, or just display it inline on the same page.
if (result != null)
{
<Label Text="@result.WelcomeText"
FontSize="16" TextColor="Color.DarkBlue" />
<Label Text="@result.CurrentAgeText"
FontSize="16" TextColor="Color.DarkBlue" />
<Label Text="@result.PlusFiveAgeText"
FontSize="16" TextColor="Color.DarkBlue" />
}
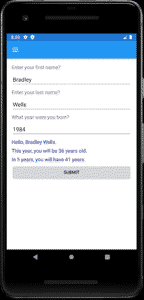
Your final Xamarin/Blazor mobile application page code may look like the following.
@using MobileBlazorApp.Models
<ContentPage>
<StackLayout Margin="new Thickness(16)">
<Label Text="Enter your first name?"
FontSize="16" />
<Entry @bind-Text="firstName" />
<Label Text="Enter your last name?"
FontSize="16" />
<Entry @bind-Text="lastName" />
<Label Text="What year were you born?"
FontSize="16" />
<Entry @bind-Text="birthYearString" />
@if (errorMessage != null)
{
<Label Text="@errorMessage" TextColor="Color.Red"
FontSize="14" />
}
else
{
if (result != null)
{
<Label Text="@result.WelcomeText"
FontSize="16" TextColor="Color.DarkBlue" />
<Label Text="@result.CurrentAgeText"
FontSize="16" TextColor="Color.DarkBlue" />
<Label Text="@result.PlusFiveAgeText"
FontSize="16" TextColor="Color.DarkBlue" />
}
}
<Button OnClick="ButtonClickHandler" Text="Submit" />
</StackLayout>
</ContentPage>
@code {
string firstName;
string lastName;
string birthYearString;
string errorMessage;
ResultModel result;
private void ButtonClickHandler()
{
if (int.TryParse(birthYearString, out int birthYear))
{
errorMessage = null;
int currentAge = DateTime.Now.Year - birthYear;
result = new ResultModel()
{
WelcomeText = $"Hello, {firstName} {lastName}.",
CurrentAgeText = $"This year, you will be {currentAge} years old.",
PlusFiveAgeText = $"In 5 years, you will have {currentAge + 5} years."
};
}
else
{
result = null;
errorMessage = "Please enter a valid year.";
}
}
}
The Bottom Line
In this tutorial, you learned how to accept user input in your Xamarin mobile applications using the Blazor programming model by creating a form and binding Entry
field values to string variables in your application. You also learned one technique for extending that functionality to allow for working with integers. Finally, you learned how to save your results to an instance of a model class which allows you to easily pass data to another component or to display results to your application's user interface.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
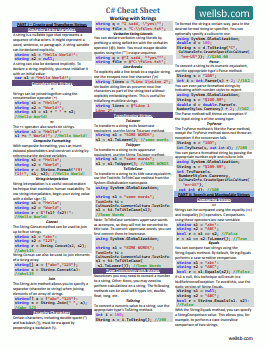
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets