In this tutorial, we will learn how to declare variables in C# and how to initialize them with values. We will also learn about different C# data types for our variables.
What is a variable?
In C#, as with any programming language, a variable simply represents an allocation of a computer's memory - like a bucket. Once we have declared our variable bucket, we can then put values into the bucket, retrieve values from the bucket, or completely change the contents of the bucket. This analogy helps us understand how we can use variables, but there is still more we need to know
Let's consider the size of container I would need in order to hold a giant boulder. I will not be able to put a boulder into a small beach pail. The beach pail is not the right size bucket for the item I want to store. In the same way, variables come in different sizes for storing different types of data. Some variables will hold numeric values, some will hold a string of alphanumeric characters, and some will hold date/time values. It is important to declare variables of an appropriate data type based on the values we wish to store in the variable.
How to Declare Variables in C#
Recall, a variable is a simply bucket in memory that we can store data in and retrieve data from. When creating a variable, we have to declare what size bucket we want to create. We will also give each variable a label that we can use to refer to that specific bucket.
To explore this topic further, we will create a new C# project in Visual Studio (File > New > Project...). We will be creating a .NET Core Console Application, so select that project type. Name your project DataTypes. If you are unsure how to create a new project, walk through our "Write your First C# Program" tutorial.
We will start by defining two variables for holding numeric values. Add the highlighted lines within your static void Main() method:
static void Main(string[] args)
{
int x;
int y;
}
With those two lines, we have declared two different variables in C#. More specifically, we have asked the .NET Core runtime to allocate space in our computer's memory large enough to hold numbers. We have not done anything yet with that space; they are just two empty buckets. This particular variable is of type Integer. I will summarize the data types we come across in the table below with a description of when you should use each type.
Now, let's assign some values to these variables. The equals sign ( = ) is known as the assignment operator, and it is what we will use to assign values to our integers.
int x;
int y;
x = 8;
y = x + 3;
If we were now to print the value of the variable y to the console, we would see that it holds a value of 11. In line 13, we told our program to set y equal to the value of variable x and then add 3 to that value, so y = x + 3;
translates into y = 8 + 3, which equals 11.
Remember, just like classes and methods, variables in C# are case-sensitive. A variable named myVariable
is different from a variable named myvariable
.
C# Data Types Example
Now, let's try a more interesting example. In this example, we will declare variables of different data types. Later, we will discuss when to use each type. Go ahead and erase the code you've already written in lines 9-13, and replace it with the following:
class Program
{
static void Main(string[] args)
{
int intVar = 10;
double doubleVar = 3.14;
char charVar = 'A';
string stringVar = "Hello from wellsb.com";
bool boolVar = true;
DateTime dateTimeVar = DateTime.Now;
Console.WriteLine("intVar = " + intVar.ToString());
Console.WriteLine("doubleVar = " + doubleVar.ToString());
Console.WriteLine("charVar = " + charVar);
Console.WriteLine("stringVar = " + stringVar);
Console.WriteLine("boolVar = " + boolVar.ToString());
Console.WriteLine("Today is " + dateTimeVar.ToString());
Console.ReadLine(); ;
}
}
The code snippet above lists examples of some of the most commonly used C# data types for variables. If you run the code, it will print the values to the console so you can see how they are stored and how they can be used. The table below summarizes these data types, but let's go ahead and learn about them from a practical perspective.
The first variable we see is of the Integer data type in Line 9. Use this type of variable when working with whole numbers, like goals scored or a number of items.
The second variable we see is of type Double (Line 10). This variable is useful when working with numbers that contain decimal values. For example, it could be used when working with rational numbers (or approximations of irrational numbers) or when making calculations involving figures with decimal values.
Next, we see the variable of data type Char (Line 11). This variable holds a single Unicode character. This could be a letter, number, or a symbol.
On Line 12, we see a String variable. This data type holds a sequence of unicode characters. Use it for storing words or sentences.
On Line 13, we initialize a Bool variable. This data type holds a value of either True or False. This is useful when coding flags, triggers, or toggles.
Finally, we have a special construct called DateTime. This variable type represents an instant in time. It is a fun structure, because there are a variety of methods we can call to perform calculations and make manipulations with DateTime variables.
Common C# Data Types
The table below is not exhaustive. There are reasons to use data types not included in this table, such as memory constraints or precision requirements. Nevertheless, this table will give you a good start as you begin to declare and initialize your first C# variables.
Type | Keyword | Description |
---|---|---|
Short | short | A whole number (no decimal values) between -32,768 to 32,767 |
Integer | int | A whole number between -2,147,483,648 to 2,147,483,647 |
Float | float | A floating point number with 7 significant digits |
Double | double | A floating point number with 15 significant digits |
Character | char | A single Unicode character (letter, number, symbol) |
String | string | A sequence of Unicode characters |
Boolean | bool | Either True or False |
DateTime | DateTime | Represents a date and time |
The Bottom Line
In this tutorial, you have learned about variables in C#. A variable is an allocation of a computer's memory that can be used for storing data. Once you have defined and initialized your variable, you can read it, change it, and use it in other parts of your program. You should now know that variables come in many different data types, and you should have an idea of when to use some of the more common C# data types.
If you have questions about C# data types or about using variables in your C# code, let me know in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
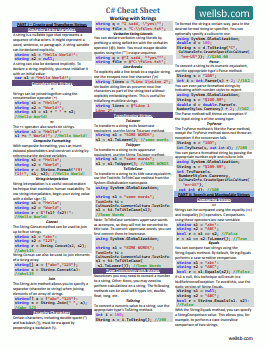
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets