If you are new to C#, the concepts of classes, methods, and properties can be overwhelming. In this tutorial, we will learn the difference between each of these and give a real-world example of how to create a C# class with methods. These definitions will help you get a good grasp of object-oriented programming.
In the previous lesson, we wrote our first C# program. In that lesson, we mentioned that class names and method names are case-sensitive. But what is the difference between a class and a method? In object-oriented programming, we can consider that everything we work with is some kind of object. As developers, we want to be able to use and manipulate these objects. To define these objects, we categorize our code blocks into different components like Classes and Methods.
What is a Class in C#?
In C#, a Class can be considered as a template or blueprint for an object. It defines which properties and functions our object needs, so we can create the object and use it. Let's consider an example where we write a program to represent a soccer team . We might create a Class called SoccerTeam. In this class, we would define fields that all soccer teams have - a name, a stadium, and number of wins, losses, and draws. Our pseudocode might look something like this:
Class SoccerTeam{
Field1 name;
Field2 stadium;
Field3 wins;
Field4 losses;
Field5 draws;
}
What is a Method in C#?
A Method is a function inside our Class that contains a series of statements. Like the Class, a Method is its own code block, but Method code blocks are located inside their respective Class code block. They can define actions to be made on the Class.
For example, we might want to create functions that allow us to add match results to our team's record or view the team's statistics. These function definitions would go inside our SoccerTeam Class code block and are called Methods. Our pseudocode might now look something like this:
Class {
Field1 name;
Field2 stadium;
Field3 wins;
Field4 losses;
Field5 draws;
Method1 AddMatch(arguments) {
Do something;
}
}
A Complete C# Class Example
Let's put it all together by studying a real example based on our SoccerTeam class.
using System;
namespace SoccerTeams
{
public class SoccerTeam
{
//Private fields
private string name;
private string stadium;
//Private fields with initial values
private int wins = 0;
private int losses = 0;
private int draws = 0;
//Property that takes private fields and computes win ratio
public double WinRatio
{
get
{
int matches = this.wins + this.losses + this.draws;
return (double)this.wins / matches;
}
}
//Method for adding matches to SoccerTeam
public void AddResult (int goalsFor, int goalsAgainst)
{
if (goalsFor > goalsAgainst) {
this.wins++;
}
else if (goalsFor == goalsAgainst) {
this.draws++;
}
else {
this.losses++;
}
}
//Override ToString() class so we can get a nice visual of what our class contains
public override string ToString()
{
return this.name + " plays at " + this.stadium + ": " + "W" + this.wins + " L" + this.losses + " D" + this.draws;
}
//Constructor which defines how the class is initialized
//Takes two arguments (name and stadium)
public SoccerTeam(string n, string s)
{
this.name = n;
this.stadium = s;
}
}
class Program
{
static void Main()
{
//Instantiate object
SoccerTeam ocsc = new SoccerTeam("Orlando City SC", "Orlando City Stadium");
//Simulate some matches
ocsc.AddResult(4, 2); //win
ocsc.AddResult(2, 2); //draw
ocsc.AddResult(1, 0); //win
ocsc.AddResult(0, 1); //loss
//Print record by calling WinRatio property.
Console.WriteLine("Win Ratio: " + ocsc.WinRatio);
//Print what is in our class
Console.WriteLine(ocsc.ToString());
//Wait for user input
Console.ReadLine();
}
}
}
In this example, we have defined a class called SoccerTeam. Within this Class, we have private Fields for the team's name, stadium, and number of wins, losses, and draws (lines 7-14). We then define a Property called WinRatio (lines 16-24) that allows us to take these private Fields, make some computations, and use the result in another class. A Property is a mechanism that allows us to read, write, or compute values of private Fields.
Next, we created a Method for adding match results to our SoccerTeam (lines 27-39). This Method takes two arguments (goalsFor and goalsAgainst) and determines whether the match was a win, a loss, or a draw for our SoccerTeam.
Creating an Instance of our Class
Remember, the Class itself is only a template for what the Object contains. We don't actually create the Object until we instantiate it in our Program class by using the new operator on Line 61. After this, we hard-code some match results and add them to our class by calling the .AddResult() method (lines 63-68).
Finally, we access the public WinRatio property and write it and some other interesting information to the console. When we run the program, we will see information about our Class Instance in the console.
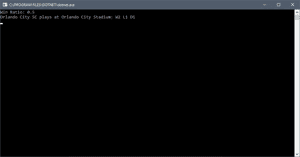
Another C# Class vs Method Example
Remember in our first C# program, we used Console.ReadLine();
and Console.WriteLine('');
. Console is actually a class defined in the .NET Core framework, and it consists of several methods and properties. ReadLine() and WriteLine() are two such methods of the Console class.
In fact, if you look closely at our first C# program example, you will see that all our code was written inside the Main method of our Program class. you were organizing your code into classes and methods without even realizing it!
The Bottom Line
In this tutorial, we have tried to answer your questions about Classes, Methods, and other common C# terms. You may not be able to write a full C# program yet, but hopefully now you have a better understanding of the difference between a Class and a Method. These concepts will become more clear as you continue to work through the tutorials. In the coming lessons, you can expect to discover more about data types, variables, and simple C# statements.
Do you have any questions about this C# Class vs Method example? Let me know in the comments.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
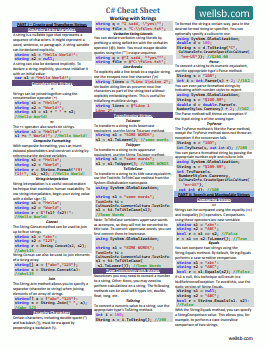
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets