In this tutorial, you will learn how to increment your C# for loop by 2 or by any other integer by modifying the iterator section of the for
statement.
Recall C# For Loop Syntax
Remember that to create a For loop in C#, the for
statement has three parts - the initializer, condition, and iterator sections.
for (int i = 0; i < length; i++) { }
In the initializer section, we declare and assign a value to the counter variable. The loop will continue to run as long as the conditions in the condition section are true. Finally, in the iterator section, we increment the counter variable.
Incrementing Counter Variable by 2
Typically, the iterator section will say i++
. This will increment our counter variable by 1 each time the loop iterates. Recall from our previous tutorial that the increment operator i++
is functionally equivalent to i = i + 1
or i += 1
.
Knowing this, we can modify the increment section of our for
statement to force our counter to use a different increment.
for (int i = 0; i < 10; i+=2)
{
Console.WriteLine(i);
}
Console.ReadLine();
In this example, our counter will increment by 2 each time the loop runs and our program will only print even numbers (0, 2, 4, 6, 8) to the console.
The Bottom Line
In this tutorial, you expanded your understanding of the classic for
loop in C#. You learned that you can customize a loop by incrementing your counter variable by non-default integers, and we gave a practical example of when this could be useful. If you have any thoughts, let me know in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
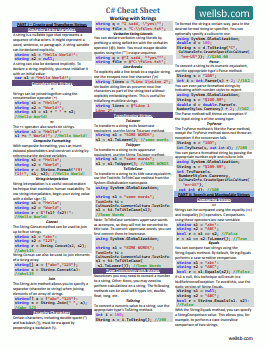
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets