In computer programming, it will often be necessary to iterate through a sequence of things in order to find a successful match. A For Loop in C# is one tool to help us with this sort of data manipulation.
C# For Loop Syntax
The syntax for creating a for
loop in C# is as follows:
for (int i = 0; i < length; i++) { }
This syntax uses the for
keyword followed by three statements in parentheses. Let's break down each of these three sections.
In the first section of our for
statement on Line 9, we see int i = 0;
. This is called the initializer section, and it is responsible for initializing our counter variable i
. This variable will only be accessible inside the loop. We can call the counter variable any name we want.
In the middle section of our for
statement, we see i < 10;
. This section is called the condition section. Our loop will iterate as long as the value of this condition is true. As soon as this condition is false, our loop will end.
The last section of our for
loop code says i++
. This is known as the iterator section. Each time our loop runs, the command in the iterator section will execute, thus incrementing the value of variable i
. The i++
syntax signifies that we want to increment the value of i
by one. Using this increment operator is functionally equivalent to i = i + 1
or i += 1
.
Notice there is no semi-colon ;
after the iterator section. The semi-colon serves as a separator between the sections of the for
statement, but it is not used after the third section.
C# For Loop Example
We can create a simple example to illustrate this useful function. Launch a new .NET Core Command Line project called ForLoop and add the highlighted code.
using System;
namespace ForLoop
{
class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i);
}
Console.ReadLine();
}
}
}
You will see the For
loop syntax on Line 9. This program will run through a loop 10 times. Each time the loop iterates, it will print one number to the console, beginning with the number 0. Once the loop has run ten times, the numbers 0-9 will all be printed to the console. At this point, the loop will end and our program will wait on Line 13 for some user input.
The Bottom Line
Iterations and loops are important parts of computer programming. There are other loops which we will visit later in our tutorial series, but the for
loop is an important tool in your C# toolbox. You will use it regularly. How do you plan to use for
loops in your programs? Let me know in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
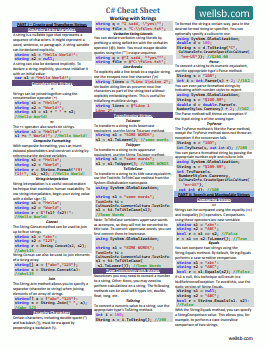
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets