In C#, enumerations, or enums, are a special data type used to limit the possible values of a given variable. Constraining data is useful for ensuring the validity of values as they are used throughout the program.
Getting Started with Enums
Suppose you were coding a To-Do application. You might create a Todo class to hold such information as the task's description, due date, and status. To ensure consistency throughout the program, it will be important to define the possible statuses. If your program supports three possible statuses, you might be tempted use three integers and create a table to define which integer corresponds to which status. You may even write this table as a code comment so programmers reviewing your code will understand the breakdown.
// 0 - Not Started
// 1 - In Progress
// 2 - Completed
While this approach will work, the meaning of each number in the source code is not readily obvious without referencing the table each time. Using an enumeration will result in more readable code, and it will help ensure you do not accidentally make a mistake later in your code by forgetting which status corresponds to which integer.
Enumerations help by allowing you to constrain the possible values to only those that you define to have meaning within the system. Behind the scenes, the default underlying data type for an enum
is still an integer. However, the enum
removes any ambiguity by directly referencing the textual equivalent instead of the numerical value.
In addition to code readability and manageability, an enumeration is more efficient than the alternative of hard-coding literal strings for each status. The underlying integer is a relatively small data type, consuming fewer memory resources than a string.
An enumeration to define possible statuses in our To-Do application might look like the following.
enum Status
{
NotStarted,
InProgress,
Completed
}
If you wish to reference this enumeration in other classes, don't forget to place the code outside of the Main()
method.
Using Enums with Classes
class Todo
{
public string Description { get; set; }
public Status Status { get; set; }
}
In the above class definition, you can see that Status is one of the properties of the Todo class. The possible values are defined in the enum
you previously created. When you instantiate an instance of the Todo class, you are able to choose from these defined values.
Todo task = new Todo { Description = "Write Blog Post", Status = Status.InProgress };
To perform an action based on the status of the Todo item, you might use a switch
statement, as you learned to use in the C# Console Menu tutorial.
switch (task.Status)
{
case Status.NotStarted:
// Do something
break;
case Status.InProgress:
// Do something else
break;
case Status.Completed:
// Yay!
break;
default:
break;
}
The switch-case
construct works beautifully with enumerations, because the resulting code is easily understandable. Recall that switch
statements are a good alternative to using multiple if-else if
statements. In this case, you can use switch-case
statements to choose to display only those tasks that are not yet completed, or to change the color of a task depending on its status.
The Bottom Line
In this tutorial, you learned about a special data type in C# known as an enumeration, or enum
. Enums help you, the developer, define valid values for a given variable. You learned how to use enums in your projects, including using them as properties in your class definitions. Finally, you learned that switch
statements and enums work very well together! As always, if this was helpful to you, let me know in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
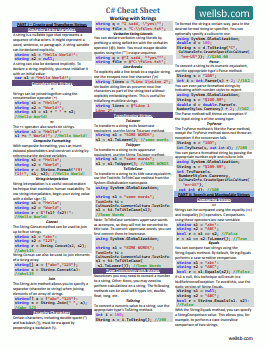
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets