In this tutorial, you will learn how to pass data as a route parameter from a Blazor component on one page to a component on another page. This is a helpful trick that enables your web apps to elegantly hand off data between various components and pages.
Using Blazor, it is easy to pass parameters to a component when the component is loaded on the same page. However, what if you want to pass a value between two different pages with different routes? One way this can be done is by using a routing parameter. (The other way is by using a singleton service. That tutorial is coming next week!)
Initial Setup
If you have not already done so in the previous tutorial, begin by creating a new Blazor WebAssembly project called ComponentParameters. In the Solution Explorer, right click Pages and navigate to Add > New Item … Select Razor Component and name it Page1.razor. Repeat the procedure and name the next component Page2.razor.
Next, open Index.razor and replace the existing content with the following code.
@page "/"
@using ComponentParameters.Pages;
<Page1 />
The using
statement corresponds to the namespace of the project plus the directory name of the file you wish to reference ComponentParameters.Pages
. Next, simply add the component in the same way you would any other html element, by enclosing its name in angle brackets <Page1 />
. This configuration will load the contents of Page1.razor when the application starts up by populating it onto the app’s home page /
.
Pass as Route Parameter
For this application, suppose you want to do some data manipulation on one page and you want to pass the result of that manipulation to a second page. The method in this tutorial allows you to pass values using route parameters in the url. This approach clearly has its limitations. You would not want to use it to pass too many parameters, as the url would get very long. You certainly would not want to use this technique to pass anything sensitive. Nevertheless, it can be useful for simple handoff scenarios.
Configure First Page
In the previous tutorial, you learned how to bind data to variables in Blazor. Using this technique, bind the value of a user’s input to a C# variable.
<h2>First Page</h2>
<input type="number" @bind="age" />
<a href="/page2/@age.ToString()" >
<button>Go to Page 2</button>
</a>
@code {
int age = 0;
}
As you can see, the input is bound to a variable field age
. When the input changes, the age
variable is also updated.
Next, create a link that will navigate to the second page.
<h2>First Page</h2>
<input type="number" @bind="age" /><br />
<a href="/page2/@age.ToString()" >
Go to Page 2
</a>
@code {
int age = 0;
}
Notice the link provided for the href
attribute includes the string
representation of the age
variable. Page 1 is complete.
Configure Second Page
The second page will need a way to handle a route with this extra parameter. To do this, use the following route directive at the top of Page2.razor.
@page "/page2/{age}"
This directive will take the {age}
parameter and look for a corresponding component [Parameter]
to assign it to. By creating a string property named Age
, Blazor will will recognize it as the appropriate parameter and assign it the value of {age}
.
@page "/page2/{age}"
<h2>Second Page</h2>
<p>You are @Age years old.</p>
@code {
[Parameter]
public string Age { get; set; }
}
Now, when you run the application, you can input a value on Page1, click the link, and it will appear on Page2. To use the original int value, simply override the OnInitialized()
method of the razor component and use int.Parse()
to get the integer value represented by the string. You might create something like the following.
@page "/page2/{age}"
<h2>Second Page</h2>
<p>You are @Age years old.</p>
<p>In 5 years, you will be @agePlusFive years old</p>
@code {
[Parameter]
public string Age { get; set; }
int agePlusFive;
protected override void OnInitialized()
{
agePlusFive = int.Parse(Age) + 5;
}
}
The Bottom Line
You already learned how to pass data between components in Blazor. In this tutorial you learned a technique for passing data between Blazor pages using routing parameters. This approach is useful when a variable set on a previous page needs to influence the content displayed on another page. How do you plan to use route parameters in your Blazor applications? Share your ideas in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
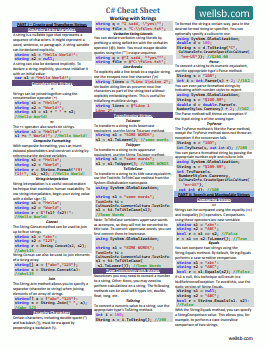
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets