One of the most useful Blazor features is the ability to pass data between your front-end DOM elements and back-end C# code. In this tutorial, you will learn techniques for binding C# variables such that they can be displayed in the browser or modified via user input in the browser.
One-way Data Binding
The first technique is a one-way binding that will attach the value of a variable to a DOM element of your website. Recall that the @
character is used to denote the beginning of C# code in a razor page or component. To display a back-end property or field within a browser element in Blazor, simply use the @
character followed by the variable name.
<h1>Greetings, @userName</h1>
@code {
string userName = "Bradley";
}
The value displayed in the h1
header will stay synced with the value of the variable userName
in the C# code. Any changes made to the value of userName
will be reflected in the website’s front-end for the user to see. Consider the following demonstration.
<h1>Greetings, @userName</h1>
<button class="btn btn-primary" @onclick="ToggleName">Change Name</button>
@code {
string userName = "Bradley";
void ToggleName()
{
if (userName == "Bradley")
userName = "Mr. Wells";
else
userName = "Bradley";
}
}
In the above example, the event-handler ToggleName()
is triggered when the buttons onclick
event is raised. When the button is clicked, the value of the C# variable userName
is changed. The new value will automatically appear in the heading of the HTML code. As you can see in this example, this technique can be used to personalize a user’s experience. It could also be used to add custom classes to DOM elements based on other data.
Two-way Data Binding
The previous technique works when variables are manipulated in the back-end C# code and you want to display them in the front-end as HTML. Suppose, however, you want to work in the other direction. In order to change the value of a C# variable based on input received by a user on your website, you will need to use two-way data binding.
To issue a two-way data bind in Blazor, use the @bind
attribute on an input
element or other form element.
<h1>Greetings, @userName</h1>
<input @bind="userName" />
@code {
string userName = "Bradley";
}
The @bind
attribute will synchronize the value of the input
field with the value of the C# variable the input is bound to, in this case userName
. Specifically, the userName
variable is updated when the input’s onchange
event is raised. The onchange
event is raised when the input element loses focus and the new value is different from the previous value.
When the user accessing the website types a new string in the input
element and then changes focus, either by tabbing to a new element or by clicking outside the element, the h1
heading will be updated.
Binding on other Events
In the above example, wouldn’t it be nicer if the heading were instantly updated as the user types? It is possible to trigger the binding after each letter is typed by using the oninput
event instead of the onchange
event. To do this in Blazor, you will use slightly different attributes.
<h1>Greetings, @userName</h1>
<input @bind-value="userName" @bind-value:event="oninput" />
@code {
string userName = "Bradley";
}
Because Blazor’s @bind
attribute automatically works in conjunction with the element’s onchange
event, you will need to manually specify that you wish to use the oninput
event to trigger the bind. In addition, you will use the @bind-value
attribute to identify the C# property or field you wish to bind to. In this case, @bind-value="userName"
and @bind-value:event="oninput"
will produce the desired results.
The Bottom Line
In this tutorial, you learned how to use Blazor to display the value of C# variables in your website’s HTML code. You also learned how to issue two-way data binding using Blazor’s @bind
attribute, which allows you to use values received by the user in your C# application . Finally, you learned how to customize data binding for different events. I would love to hear in the comments about the web applications you will build using these techniques.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
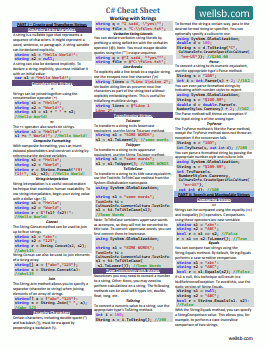
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets