If your C# web app targets .NET Core 3.1 or greater, you can use the new IdentityModel.AspNetCore
package to easily manage access tokens provided by an IdentityServer4 authentication server. Let’s get started.
In this Blazor tutorial series
- Part 1: Blazor with Web API Solution Structure
- Part 2: Consume API protected by IdentityServer4
- Part 3: Cache IdentityServer4 API Access Token
- Part 4: The Easy Way
Install Nuget Package
Instead of creating a custom token management solution for your app and adding a custom caching solution like we did in Part 2
and Part 3 of this series, you can make access token management completely transparent so you can focus on the more important things!
Open the BlazorContacts solution and load the BlazorContacts.Web project. Start by installing the latest version of the new IdentityModel.AspNetCore
Nuget package.
Install-Package IdentityModel.AspNetCore
Configure ApiService
With this technique, you will not need to manually fetch an access token. In fact, your ApiService.cs file will look as though you are just accessing any public API, not one protected by IdentityServer4 authentication. Your GetContactsAsync()
method will look like it did in Part1.
public async Task<List<Contact>> GetContactsAsync()
{
var response = await _httpClient.GetAsync("api/contacts");
response.EnsureSuccessStatusCode();
using var responseContent = await response.Content.ReadAsStreamAsync();
return await JsonSerializer.DeserializeAsync<List<Contact>>(responseContent);
}
Be sure to remove any references to the ApiTokenCacheService
you injected as a dependency in the previous tutorial, as well. This simple technique will also handle caching on its own.
Enable Access Token Handler
To enable automatic access token management, you simply need to add a couple lines to the Startup.cs file of the client you have granted API access to. You do not need to change anything in the BlazorContacts.Auth server configuration or the BlazorContacts.API.
Open Startup.cs of BlazorContacts.Web and locate the ConfigureServices()
method. If you have been following along the other lessons, comment out the line that adds the ApiTokenCacheService
to the services collection. It is no longer needed.
You should have already added a typed HttpClient to be used for API calls. To enable the automated token handler, simply chain the AddClientAccessTokenHandler()
to that definition.
services.AddHttpClient<Services.ApiService>(client =>
{
client.BaseAddress = new Uri("http://localhost:5001");
})
.AddClientAccessTokenHandler();
Then, configure the token manager by providing the client credentials to the token management services. You must provide the token endpoint, which corresponds to the address of the BlazorContacts.Auth server. The ClientId
and ClientSecret
are the unique ID and secret key you assigned to your authorized client in Config.cs.
services.AddAccessTokenManagement(options =>
{
options.Client.Clients.Add("auth", new ClientCredentialsTokenRequest
{
Address = "http://localhost:5000/connect/token",
ClientId = "blazorcontacts-web",
ClientSecret = "thisismyclientspecificsecret"
});
});
That’s all! Your Blazor web app will automatically negotiate an access token when making an API call to your IdentityServer4 protected API
The Bottom Line
In part 1 of this series, you learned how to correctly consume an external web API by using the HttpClientFactory. In part 2, you learned how to restrict access to your API to only authorized applications, and you learned how to request an access token from an authentication server in Blazor. In part 3, you learned a technique for caching the access token for a period of time so you no longer had to request a new one for each API call. And finally, in part 4, you learned an easy way to automatically manage access tokens behind the scenes.
Source code for this project is available on GitHub.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
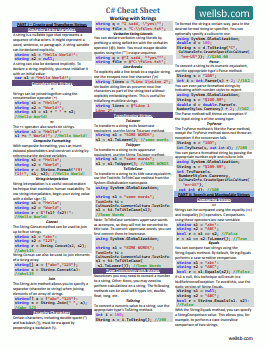
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets