Toast notifications are timed alerts that appear at the bottom of the screen and provide simple feedback to the user about an operation in a small alert. They are automatically dismissed after a configurable duration of time. In this tutorial, you will learn how to use the .NET MAUI Community Toolkit library to create and display toast notifications in your .NET MAUI app.
Getting Started
To get starting using toast notifications in a .NET MAUI app, install the .NET MAUI Community Toolkit library from NuGet. You can do this by using the Package Manager Console in Visual Studio. Run the following command:
Install-Package CommunityToolkit.Maui
This will add a reference to the CommunityToolkit.Maui assembly to your project. You also need to add the following namespace to your C# code file:
using CommunityToolkit.Maui.Alerts;
This will allow you to access the Toast class and its methods.
Creating and Displaying a Toast
To create a toast, you can use the static method Toast.Make()
, which takes a string parameter for the text that will be displayed in the toast. You can also optionally specify the duration and the font size of the toast. For example:
string text = "Free C# tutorials at wellsb.com";
ToastDuration duration = ToastDuration.Short;
double fontSize = 14;
var toast = Toast.Make(text, duration, fontSize);
The ToastDuration
enumeration defines two values: Short
for 2 seconds and Long
for 3.5 seconds. The default value is Short
. The default font size is 14.
To display the toast, you can use the Show()
method of the toast object. This method returns a task that you can await or ignore. For example:
await toast.Show();
The following screenshot demonstrates how this toast would appear on an Android device.
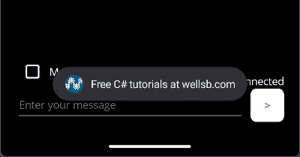
The Bottom Line
In this tutorial, you learned how to use the .NET MAUI Community Toolkit library to create and display toast notifications in your .NET MAUI app. Toast notifications are useful for providing simple feedback to the user about an operation in a small alert.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
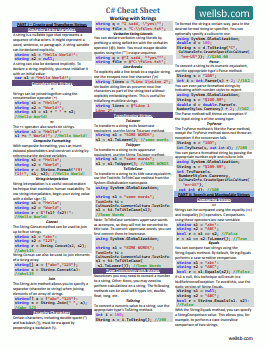
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets