Tweetinvi is a C# wrapper library for interfacing with the Twitter API. This tutorial will teach how you to use Tweetinvi to develop .NET applications that can read tweets or write them on behalf of a user.
Getting Started
To get started, you will need a Twitter API Key and API Secret. You can obtain these for your app from the Twitter Developer Portal. To perform actions on behalf of a user, you will also need to generate an access token and secret. Once you have these, securely save them as an environment variable in your development environment.
Now you are ready to begin coding. Start by creating a new C# Console Application targeting .NET 5.0. Install the TweetinviApi Nuget package.
Install-Package TweetinviAPI
Initialize Twitter Client
For this tutorial, we will write a helper class that we can use to interface with the Twitter API. The public helper class will contain a private TwitterClient
instance. Add a new class to your project called TwitterHelper.
public class TwitterHelper
{
private TwitterClient _twitterClient { get; set; }
}
Import the following Tweetinvi namespaces.
using Tweetinvi;
using Tweetinvi.Models;
To initialize _twitterClient
, overloaded a constructor for this helper class. The constructor should accept the parameters expceted by TwitterClient in Tweetinvi, namely the API keys and access tokens you generated from the Twitter Developer Portal.
public class TwitterHelper
{
private TwitterClient _twitterClient { get; set; }
public TwitterHelper(string apiKey, string apiSecret, string accessToken, string accessSecret) {
_twitterClient = new TwitterClient(apiKey, apiSecret, accessToken, accessSecret);
}
}
Now, in Program.cs, you can initialize an instance of the TwitterHelper object.
var twitterHelper = new TwitterHelper(
Environment.GetEnvironmentVariable("TwitterApiKey"),
Environment.GetEnvironmentVariable("TwitterApiSecret"),
Environment.GetEnvironmentVariable("TwitterAccessToken"),
Environment.GetEnvironmentVariable("TwitterAccessTokenSecret"));
The Environment.GetEnvironmentVariable()
method will retrieve the value for the corresponding key from your system's environment variables. The key names provided should match those of the environment variables you used to store your API key data and access token values.
We will be using async
methods in this tutorial, so change the signature of static void Main(string[] args)
to static async Task Main
Search and Fetch Tweets
You are now ready to begin working with your TwitterHelper object. Write a method that will accept a query string and return an array of tweets matching the query. We will use V1 of the Twitter API for this portion of this example.
public async Task<ITweet[]> SearchTweetsAsync(string query)
{
if (_twitterClient == null)
return null;
try
{
return await _twitterClient.Search.SearchTweetsAsync(query);
}
catch (Exception)
{
throw;
}
}
This method will ensure that the TwitterClient
has been initialized, and then it will attempt to return an array of Tweets that match the search query.
To print the latest tweets that contain the hashtag #dotnet, you could use this method in Program.cs as follows.
var tweets = await twitterHelper.SearchTweets("#dotnet");
foreach(var tweet in tweets)
{
Console.WriteLine(tweet.Text);
}
Console.ReadLine();
Post a Tweet for User
For this portion of the tutorial, be sure your registered Twitter application in the Twitter developer portal has permission to both read and write.
Write a new method in TwitterHelper.cs that will post a provided text as a tweet.
public async Task<ITweet> TweetAsync(string tweetText)
{
if (_twitterClient == null)
return null;
try
{
return await _twitterClient.Tweets.PublishTweetAsync(tweetText);
}
catch (Exception)
{
throw;
}
}
To use this method of our TwitterHelper
object, simply pass it the text you wish to tweet.
var tweet = await twitterHelper.TweetAsync("This is a tweet from wellsb.com");
if (tweet != null)
Console.WriteLine($"Tweet sent successfully:\n{tweet.Text}");
The Bottom Line
In this tutorial, you learned how to use the TweetinviAPI library in order to incorporate Twitter API capababilities into your .NET application. You wrote a helper class to handle Twitter API functions, and you initialized an instance of the class through an overloaded constructor. Finally, you wrote one method for searching tweets given a query string and another method for sending a tweet on behalf of the authorized user.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
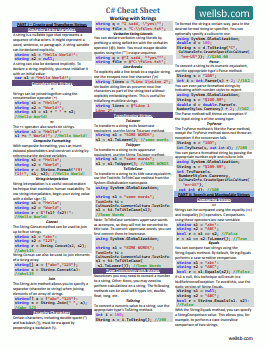
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets