In the world of C# programming, one of the essential concepts is initializing the properties of a class. Properties are named members of a class that provide a flexible mechanism to read, write, or compute the values of private fields. In this tutorial, we will explore the C# init
operator, when to use it, and compare it with other approaches to property initialization.
What is the C# Init Operator?
Introduced in C# 9.0, the init
operator is a new keyword that allows you to set the value of a property during object initialization. It is designed to provide more control over the immutability of objects, ensuring that once a property is set, it cannot be changed. The init
operator can be used with both auto-implemented and manually implemented properties.
Here's an example of how the init
operator is used with an auto-implemented property:
public class Person
{
public string Name { get; init; }
}
In this example, the Name
property can only be set during object initialization, like this:
Person person = new Person { Name = "John Doe" };
Once the property is set, it cannot be changed:
person.Name = "Jane Doe"; // This will result in a compile-time error
When Should You Use the Init Operator?
The init
operator is best used when you want to create immutable objects, which are objects whose state cannot be changed after they are created. Immutability is a desirable property in many scenarios, especially in multi-threaded or concurrent programming, as it can help prevent bugs and make the code more maintainable.
Some common use cases for the init
operator include:
- Value objects: Objects that represent a value, such as a date, time, or currency, where the state should not change after creation.
- Data transfer objects (DTOs): Objects used to transfer data between different layers of an application, where the state should remain consistent.
- Configuration objects: Objects that hold configuration settings, where the state should not change after the application starts.
Comparing Init Operator with Other Property Initialization Approaches
There are several ways to initialize properties in C#. Let's compare the init
operator with some of the other common approaches:
1. Public Setter
The most straightforward way to initialize a property is by using a public setter:
public class Person
{
public string Name { get; set; }
}
However, this approach has some drawbacks:
- The property can be changed at any time, making it mutable.
- It doesn't provide any control over when or how the property can be set.
2. Constructor
You can also initialize properties using a constructor:
public class Person
{
public string Name { get; private set; }
public Person(string name)
{
Name = name;
}
}
This approach provides some control over property initialization, but it has some limitations:
- The properties must be set in a specific order, as defined by the constructor parameters.
- It can become verbose and hard to maintain when there are many properties to initialize.
3. Readonly Properties
Another way to create immutable properties is by using private readonly
backing fields:
public class Person
{
private readonly string _name;
public string Name => _name;
public Person(string name)
{
_name = name;
}
}
This approach ensures that the property is immutable, but it has some downsides:
- It requires manually implementing the property with a backing field, which can be less concise and harder to maintain.
- It doesn't provide the same flexibility as the
init
operator for object initialization.
The Bottom Line
The C# init
operator is a powerful and flexible way to create immutable properties in your classes. It provides a balance between control and simplicity, making it a valuable addition to any C# developer's toolkit. In this tutorial, you learned when and how to use the init
operator, and you saw it how compares to other property initialization approaches.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
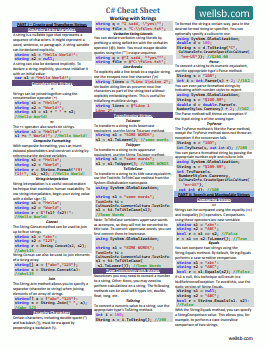
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets