In this tutorial, we will write a string manipulation program to reverse any string in C#. Using a char array and helper methods, we will be able to print a string backwards.
In previous tutorials, you have learned how to declare and initialize arrays and you know how to use loops to iterate through the elements of an array. We will build on these lessons and learn a useful technique for manipulating strings using arrays.
Convert String to Array of Chars
Suppose we have a string, "ABCDEFGHIJKLMNOPQRSTUVWXYZ" and we want to reverse the order of the letters, so the alphabet is written backwards. The last letter will appear first and the first letter will appear last.
To do this, we will split our string into an array of single characters, "A" "B" "C" and so forth. Once we have an array consisting of all the letters of our string, we will be able to reverse the array, and then convert our character array back into a string.
First, to convert a string to char[]
array, we will take advantage of a helper method available in the .NET Core framework.
string myString = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
char[] charArray = myString.ToCharArray();
On Line 2, we have declared a new char[] array and initialized its values. The ToCharArray()
helper method in C# allows us to automatically convert a string to an array of characters. It will take the string and add each character of the string to a char[] array.
Reverse Array in C#
Now that the individual characters of our original string are collected into a char[] array, we can use the Reverse()
utility method of the Array class to reverse the order of the array.
string myString = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
char[] charArray = myString.ToCharArray();
Array.Reverse(charArray);
On Line 4, we have passed our charArray to the Array.Reverse()
method. This method reverses the order of the elements in our array. Our array of characters is now in the order we want; we just need a way to concatenate each of these individual characters back into a string.
Convert Char[] Array to String
There are two ways to convert an array of characters back into a string. First, we will use a foreach loop. In the body of the loop, we will iterate through charArray and append each character to a new string.
string myString = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
char[] charArray = myString.ToCharArray();
Array.Reverse(charArray);
string newString = "";
foreach (char tempChar in charArray)
{
newString += tempChar;
}
//Result: newString = "ZYXWVUTSRQPONMLKJIHGFEDCBA"
On Line 6, we have declared a new string variable and initialized it is an empty string. This is the string we will use to hold the value of our reversed string.
On Line 8-11, you see our foreach
loop. This loop will iterate through each char
in our char[] array called charArray. Remember, charArray now holds the characters of our original string in reverse order. We will use the concatenation assignment operator +=
to append the current character to our newString variable. After the loop completes, newString will hold the value of our original string printed backwards.
In C#, there is actually a simpler way to convert a char[] array into a single string. We can replace the foreach
loop from Line 8-11 with a single line of code using a special method of the String Class. Just like we discovered the Reverse()
method of the Array class, C# also has a String class with useful methods. We can invoke the Concat()
method of the String class to easily convert an array of characters to a string. Replace line 6-11 with the following line of code:
string newString = String.Concat(charArray);
A Complete Example
You could use this technique to write a program to reverse any string a user enters. Recall from a previous tutorial how we used [Console.ReadLine()](/csharp/beginners/get-user-input-in-csharp-with-console-readline/)
to get a user's input. We will save the entered string to a variable, and use the techniques we learned in this lesson to convert the string to a char[] array, reverse the array, and add each element of the reversed array to a new string variable. We can then print the backwards string to the console.
using System;
namespace StringToCharArrayMethods
{
class Program
{
static void Main(string[] args)
{
Console.Write("Enter the string you want to reverse: ");
string myString = Console.ReadLine();
DisplayResult(ReverseString(myString));
Console.ReadLine();
}
private static string ReverseString(string message)
{
char[] charArray = message.ToCharArray();
Array.Reverse(charArray);
return String.Concat(charArray);
}
private static void DisplayResult(string message)
{
Console.WriteLine($"Your backwards string is: {message}");
}
}
}
In this example, I have taken the code we have written and organized it into methods. Each method is simple and does one thing. For example, there is a method whose job is to actually reverse the string and a method whose job is to display the results to the console. You will also notice the $
symbol on Line 26. This indicates that I am using the String Interpolation technique to include the variable message
in the returned string.
The Bottom Line
In this tutorial, you learned how to reverse a string in C#. We used the ToCharArray()
to convert a string to an array of characters. We, then, used the Array.Reverse()
method to reverse the order of the elements in the char[] array. Finally, we used the String.Concat()
method convert an array of characters to a string. How will you use this technique in your applications? Let me know in the comments.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
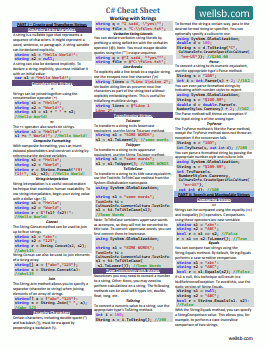
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets