In this tutorial, we will write a string manipulation example to trim all whitespace from a string in C#. We will use a helper method to remove all whitespace and print the modified result to the console.
In the previous tutorial, we wrote a console application that took a user's input and printed their string backwards. In this example, we will write a similar string manipulation program that will remove white space from the string entered by the user.
Application Outline
Get started by creating a new C# console application. For this example, we want to allow a user to enter any string. We will organize our code into methods. We will create one method whose purpose is to remove all whitespace from the string, and another method to write the modified string to the console.
Our static void Main()
method will be as follows:
static void Main(string[] args)
{
Console.Write("Enter the string you want to modify: ");
string myString = Console.ReadLine();
DisplayResult(RemoveWhiteSpace(myString));
Console.ReadLine();
}
Line 3 prints instructions for the user, and Line 4 waits for the user to enter a string and then captures it as a variable. On Line 6, you will see two methods that have not yet been defined DisplayResult
and RemoveWhiteSpace
.
Remove Whitespace from String
RemoveWhiteSpace
should accept a string
variable as an argument, and it should return a string that can be used by our program. Define the RemoveWhiteSpace
method:
private static string RemoveWhiteSpace(string message){ }
This definition allows us to pass a string to the method and reference the string internally using the variable name message
. In this method, we first need to remove all spaces from the variable message
. Then, we need to return the modified string. We can do all this with a single line of code.
private static string RemoveWhiteSpace(string message)
{
return message.Replace(" ", "");
}
On Line 3, we have used the Replace()
helper method. This method takes two arguments (of type char
or string
), and it replaces all instances of the first char or string with the second one. In this example, it will replace all whitespace characters " "
with an empty string ""
.
Display Results
Now, we need to define a method for printing the modified string to the console. This method will accept a string as an input argument, but it will not return a value.
private static void DisplayResult(string message)
{
Console.WriteLine($"Your modified string is: {message}");
}
On Line 3, you will notice the $
character before the string. This String Interpolation technique allows me to insert a variable directly in the string declaration by enclosing its name in curly braces, {message}
.
A Complete Example
using System;
namespace TrimWhiteSpace
{
class Program
{
static void Main(string[] args)
{
Console.Write("Enter the string you want to modify: ");
string myString = Console.ReadLine();
DisplayResult(RemoveWhiteSpace(myString));
Console.ReadLine();
}
private static string RemoveWhiteSpace(string message)
{
return message.Replace(" ", "");
}
private static void DisplayResult(string message)
{
Console.WriteLine($"Your modified string is: {message}");
}
}
}
The Bottom Line
In this tutorial, you learned how to trim whitespace from a string in C#. You used best practices to write a complete console application with code organized into simple methods. Your program takes a string input, removes all spaces using the Replace()
helper method, and writes the resulting string back to the console. If you appreciate this tutorial, let me know how it helped you in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
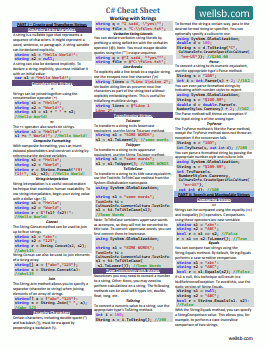
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets