The Language Integrated Query (LINQ) syntax is a powerful way to work with collections in C#. In this tutorial, you will learn how to use LINQ to filter data from C# lists. You will also learn how to chain LINQ queries together in order to return precisely the data you are interested in.
Initial Setup
The initial setup for this tutorial will be similar to that of the previous tutorial. We will continue the example of cataloging the books in a library. Start with the code below. You will see that we have already created a Book class (Line 27-39) that will hold the Title, Author, and Publication Date of each book. In addition, we have initialized a collection of ten books, specifically a List<Book>
on Lines 11-23. Notice, also, that we have already referenced the namespaces for System.Linq
and System.Collections.Generic
(Lines 2-3). If you have questions about any of this, feel free to review previous tutorials.
using System;
using System.Linq;
using System.Collections.Generic;
namespace IntroducingLINQ
{
class Program
{
static void Main(string[] args)
{
List<Book> bookList = new List<Book>()
{
new Book("Les Miserables", "Victor Hugo", 1862),
new Book("L'Etranger", "Albert Camus", 1942),
new Book("Madame Bovary", "Gustave Flaubert", 1857),
new Book("Le Comte de Monte-Cristo", "Alexandre Dumas", 1844),
new Book("Les Trois Mousquetaires", "Alexandre Dumas", 1844),
new Book("Candide", "Voltaire", 1759),
new Book("Notre-Dame de Paris", "Victor Hugo", 1831),
new Book("Vingt mille lieues sous les mers", "Jules Verne", 1872),
new Book("Le Tour du monde en quatre-vingts jours", "Jules Verne", 1869),
new Book("Voyage au centre de la Terre", "Jules Verne", 1864)
};
}
}
class Book
{
public string Title { get; set; }
public string Author { get; set; }
public int PubDate { get; set; }
public Book(string title, string author, int pubDate)
{
Title = title;
Author = author;
PubDate = pubDate;
}
}
}
LINQ Method Syntax: Filter C# Lists
In the previous tutorial, you learned two ways to work with LINQ. In this tutorial, we will focus only on the second approach, the LINQ Method syntax.
Suppose we want to list only those books which are written by author Jules Verne. C# collections allow the use of a Where()
method, which can be used to filter the collection based on specified conditions. Within the Where()
method, we can use a lambda expression to define our desired conditions for each given instance in the collection.
var subList = bookList.Where(p => p.Author == "Jules Verne");
Console.WriteLine($"{subList.Count()} books match your search criteria:");
foreach (Book book in subList)
{
Console.WriteLine($" {book.Author} - {book.Title} ({book.PubDate})");
}
Console.ReadLine();
This code fragment should be added before the end of your static void Main()
method, but after the initialization of bookList
. In this example, you will see a lambda expression on Line 25 which checks the author's name. Only those instances in the collection where the lambda expression resolves to true will be returned and added to a new list, subList
. In essence, Line 25 tells our program to execute the following logic: For any given item in a collection, check if it matches a specified criteria. If it does, add it to the new collection.
You can use logical operators within the lambda expression in order to further restrict or broaden the search. If you want, for example, to include books written by Jules Verne or Victor Hugo, you could combine queries using the logical OR operator ||
.
var subList = bookList.Where(p => p.Author == "Jules Verne" || p.Author == "Victor Hugo");
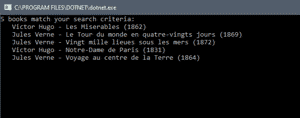
Filtering and Sorting
The filtered list above is nice, but it is not well organized. Now that we have a filtered results list, we might want to sort it to ensure that books by the same author are grouped together. Fortunately, there is a way to chain together multiple methods in C#. This can be done to combine our filtered list with the sorting method we learned previously.
You can chain together the Where()
method from Line 25 with the OrderBy()
method using the .
token.
var subList = bookList.Where(p => p.Author == "Jules Verne" || p.Author == "Victor Hugo").OrderBy(p => p.Author);
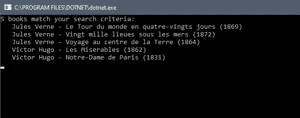
The resulting list is an improvement, but is there a way to group the books by author, and then sort by publication date? LINQ offers a way to apply a secondary sort which we can use to achieve our desired result. To do this, chain a ThenBy()
method to the query. Your code will look like:
var subList = bookList.Where(p => p.Author == "Jules Verne" || p.Author == "Victor Hugo")
.OrderBy(p => p.Author)
.ThenBy(p => p.PubDate);
Now our LINQ query filters our collection of books down to only those written by either Victor Hugo or Jules Verne (Line 25). Next, it orders the resulting list by author (Line 26). Finally, it applies a secondary ordering by publication date (Line 27). This should produce an output similar to the following:
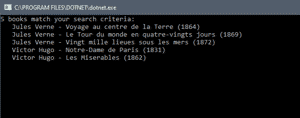
The Bottom Line
In this tutorial, you learned how to filter a C# collection using a LINQ query. Specifically, you learned how to use the Where()
query to filter a List<T>
according to specified parameters. You also learned how to chain methods together, and you used this technique to add a primary sort OrderBy()
and a secondary sort ThenBy()
to a filtered list.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
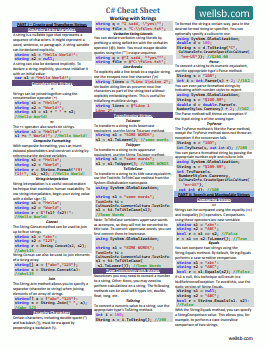
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets