In this tutorial, you will learn how to exit a For loop in C#. You can break a For loop using the break;
statement.
Breaking a For Loop
By now, you understand the syntax of a For loop in C#.
for (int i = 0; i < length; i++) { }
This loop will run as long as long as the conditions in the conditions section (i < length
) are true. Suppose, however, that you want your loop to run 10 times, unless some other conditions are met before the looping finishes.
If you want to break out of your loop early, you are in luck. The break;
statement in C# can be used to break out of a loop at any point.
using System;
namespace ForLoop
{
class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i);
if (i == 7)
{
Console.WriteLine("We found a match!");
break;
}
}
Console.ReadLine();
}
}
}
In this example, we have added an [if](/csharp/beginners/csharp-if-else-if-conditional-statements/)
statement inside our loop (Line 12) to check for a specific condition. If that condition is met, the program will execute the code inside the code block on Lines 13-16. Line 15 is part of that code block and contains the break;
statement. Once this statement is executed, the program will break out of the for
loop and jump to Line 18.
With the simple break;
statement, you can exit your loop before it is scheduled to end and continue with the next line of code after the loop code block.
The Bottom Line
In this tutorial, you learned how to break out of a for
loop. This is useful when using a loop for data manipulation or for finding matching items. If you have any questions, let me know in the comments!
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
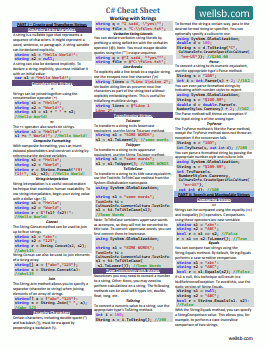
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets