To read a text file in C#, you will use a StreamReader object. In this tutorial, you will learn how to use StreamReader to read the contents of an existing file.
For this tutorial, you will start by creating a blank file on your Desktop called ReadFile.txt
. Next, copy and paste the following five lines to the file and save it.
1. Learn C# online at wellsb.com
2. Free C# tutorials
3. C# tutorials for beginners
4. Learn to code in C#
5. Become an expert in C# programming
Using StreamReader to Read a File
Create a new C# project and include the System.IO directive. The System.IO directive allows you to reference such objects as StreamReader and StreamWriter.
using System;
using System.IO;
The file that we want to read is located on the Desktop. We can define the path to this file, by providing the directory and filename to the Path.Combine()
method. We will save the file path as a string variable and pass it to the StreamReader object.
string filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.Desktop), "ReadFile.txt");
using (StreamReader inputFile = new StreamReader(filePath))
{
Console.WriteLine(inputFile.ReadToEnd());
}
As you can see, the code for reading from a text file is similar to the process we used to write to a text file using StreamWriter. We create a StreamReader object called inputFile
on Line 12, and we declare a new instance of that object by passing it the path of the text file we want to read.
Recall that the using
statement on Line 12 is to ensure that the StreamReader object is correctly disposed of, even if an error occurs. We will learn more about error handling later in this tutorial.
On Line 14, we use the ReadToEnd()
method of the StreamReader object to read all the contents of the text file. This method returns a string value. Since we just opened the document, we can think of the cursor or pointer being at the the beginning of the document. The ReadToEnd()
method reads from the current position in the document to the end of the document.
In this case, we can expect to see all five lines of our text document printed to the console. The output of our program should look like the following:
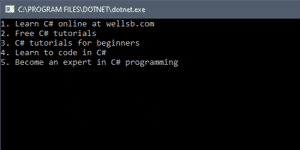
It is worth noting that StreamReader also includes methods for reading individual characters Read()
and for reading line-by-line ReadLine()
. In the next tutorial, you will learn how to use StreamReader to read a specific line in a text file.
Try/Catch Error Handling
Suppose the file that we want to read does not exist? As the program is currently written, if the file does not exist, our application will throw an exception and crash. This is not a pleasant end-user experience. When designing a program, it is important to ensure that these types of potential errors are appropriately handled.
For this exercise, we will learn about try
/catch
error handling. Specifically, we want our application to try to open the file for reading. If the file does not exist, we should present a friendly error message to the user.
To use try
/catch
blocks, consider the following template.
try
{
//Code goes here
}
catch (IOException)
{
//Error handling goes here
}
In our example, we will place our file I/O into a try
block, and return any error messages in catch
blocks. Our program might look like the following:
try
{
string filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.Desktop), "ReadFile.txt");
using (StreamReader inputFile = new StreamReader(filePath))
{
Console.WriteLine(inputFile.ReadToEnd());
}
}
catch(Exception e)
{
Console.WriteLine("There was an error reading the file: ");
Console.WriteLine(e.Message);
}
Console.ReadLine();
On Line 10 of this example, we have used a general catch
block that handles all exceptions, but it is good practice to handle specific errors. For example, the StreamReader file I/O operation could throw a FileNotFoundException
when the file is not found. It could throw a DirectoryNotFoundException
when the directory is not found. Or, it could throw an IOException
if the file cannot be opened for other reasons. Our program could provide custom error messages for each of these three exceptions by using multiple catch
statements as part of a try-catch-catch-catch block.
The Bottom Line
In this tutorial, you learned how to use the StreamReader
object to read a text file in your C# applications. This could be useful for reading a settings file or for restoring default settings from a file. You also learned how to do basic error handling. Specifically, you learned how to catch exceptions using a try
/catch
block. In the next tutorial, you will learn how to use StreamReader to read a specific line from a text file.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
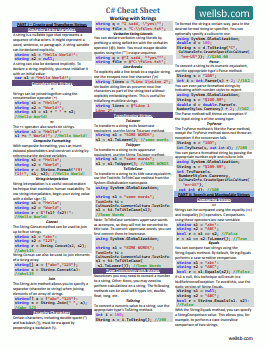
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets