For a given class in C#, you may need different ways to pass arguments to it. You can do this by overloading a constructor. Overloaded constructors enable you to initialize objects of the same class in different ways - for example, by passing in different numbers of initial parameters.
What is Constructor Overloading?
In this tutorial, you will learn about constructor overloading by working through an example. Suppose you want to keep track of all the books you have in your library. In C#, you might create a Book class that contains information such as the book's title, author, publisher, and publication date. For each book in your library, you might want to store information in a database.
When you create an instance of your object, sometimes you may only have the book's title and author. Other times, you may have the title, author, publisher, and publication date. You can create different overloaded versions of the Book class constructor to allow for both these scenarios.
What is a Constructor
A constructor is simply a method that allows you to execute code at the moment that a new instance of a class is created. Below is a basic declaration for a Book class. (Need a refresher about C# Classes and Methods?) The Book class contains the four properties we are interested in - Title, Author, Publisher, and PubDate.
class Book
{
public string Title { get; set; }
public string Author { get; set; }
public string Publisher { get; set; }
public int PubDate { get; set; }
}
When you are ready to create an instance of Book, you will use the new
keyword followed by the name of the class and a method invocation operator ()
.
Book myBook = new Book();
When you create a new instance of a class, you are calling a method that puts the object into a valid state. This method is called a constructor. A constructor is generally used to initialize the values of the properties of a given object.
How to Overload a Constructor
If you haven't defined any constructors, a default constructor will automatically be created for you at compile time. The implicit default constructor has no input parameters and no method body. It is equivalent to the following.
public Book()
{
}
To create an overloaded constructor in C#, you will change the signature of the class. In other words, you will simply change the number or data type of the constructor's input parameters.
public Book(string title, string author)
{
Title = title;
Author = author;
}
public Book(string title, string author, string publisher, int pubDate)
{
Title = title;
Author = author;
Publisher = publisher;
PubDate = pubDate;
}
In the above code fragment, I have created two overloaded versions of my Book class constructor. The first, on Line 13, takes two arguments - title and author. I can create a Book object using this class constructor as follows:
Book myBook = new Book("Les Miserables", "Victor Hugo");
The second constructor, beginning on Line 19, sets initial values for all four parameters. Here is how you would create an instance of the Book class using this version of the class constructor.
Book myBook = new Book("Les Miserables", "Victor Hugo", "Lacroix, Verboeckhoven & Cie", 1862);
C# Overloaded Constructors Example
My complete Book class has four read-write parameters, and two overloaded constructors. This gives me flexibility with respect to how I want to create my object instances. Here is the complete class code:
class Book
{
public string Title { get; set; }
public string Author { get; set; }
public string Publisher { get; set; }
public int PubDate { get; set; }
public Book(string title, string author)
{
Title = title;
Author = author;
}
public Book(string title, string author, string publisher, int pubDate)
{
Title = title;
Author = author;
Publisher = publisher;
PubDate = pubDate;
}
}
The Bottom Line
In this tutorial, you learned about C# class constructors and how to overload constructors. Overloading constructors in C# enables you to use a single class definition and instantiate objects in different ways. It allows you to define valid states for your class and set initial values for the properties of a given object at the moment the instance is created. If you want to be able to create an object based on any number of the properties of a class, you can create an overloaded constructor method to accomplish that.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
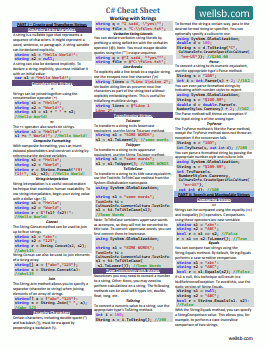
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets