If you are using Statiq.Web with npm
packages, you may be wondering how to copy files from the node_modules
directory to your project's output
folder. Fortunately, Statiq has you covered. In this tutorial, you will learn how to build SASS Bootstrap (from .scss files) and include them in your project.
Why compile from SASS?
It is easy to include cached versions of Bootstrap in your web project by using BootstrapCDN, so why would you want to compile it with Statiq.Web's built-in SASS compiler? The short-answer is that compiling from SASS is the easiest way to customize Bootstrap, such as by adding themes or button variants.
Copy files from node_modules folder
Later in this tutorial, you will learn a technique for doing this without adding any new pipelines to your project. For now though, let's walk through the processing of reading, processing, and writing files pulled in by a package manager like npm
.
Suppose you have the following in your npm package.json file. (Bonus: If you modify your npm Configuration file from within Visual Studio, you don't have to configure npm build commands because the packages will be added when you save the package.json.)
{
"version": "1.0.0",
"name": "asp.net",
"private": true,
"devDependencies": {
"bootstrap": "latest"
}
}
Since your npm packages are in the node_modules folder instead of the input folder, Statiq will not automatically find and copy them to the output folder. To tell Statiq about these files, simply modify the bootstrapper.
static async Task<int> Main(string[] args) =>
await Bootstrapper.Factory
.CreateWeb(args)
.BuildPipeline(
"Bootstrap",
builder => builder
.WithInputReadFiles("../node_modules/bootstrap/scss/**/{!_,}*.scss")
.WithProcessModules(
new Statiq.Sass.CompileSass().WithCompressedOutputStyle(),
new SetDestination(Config.FromDocument(doc => new NormalizedPath($"./assets/scss/{doc.Source.FileName.ChangeExtension(".css")}")), true))
.WithOutputWriteFiles()
)
.RunAsync();
With this new "Bootstrap" pipeline, all of Bootstrap's .scss files that don't begin with an underscore (_
) will be read from their location in the node_modules folder. They will be compiled using Statiq.Web's built-in SASS compiler, and the resulting files will be compressed.
By default, Statiq places files in the output folder according to its relative path in the input folder. Since these files did not come from the input folder, you can set the desired destination for the compiled files in the output folder using the SetDestination
module. Now, when the output files are written, they will be placed in the output/assets/scss/ directory.
You can now include them in your layout file, such as _layout.cshtml.
<link rel="stylesheet" href="/assets/scss/bootstrap.css">
Compile from npm the easy way
Now that you know how to work with a Statiq pipeline's input, process, and output phases, you will be happy to know this can be done with default bootstrapper configuration.
static async Task<int> Main(string[] args) =>
await Bootstrapper.Factory
.CreateWeb(args)
.RunAsync();
The trick is to create a .scss file within the input folder and import the bootstrap files from the node_modules folder. For example, create a file called bootstrap.scss and place it in the input/assets/scss/ folder. Now, add the following one line to that document.
@import "../node_modules/bootstrap/scss/bootstrap";
Now, when you run the program, you will end up with a full bootstrap.css file in your project's output/assets/scss directory.
Customize Bootstrap themes
But why stop here? Let's take full advantage of what the world of SASS has to offer. You can easily add custom Bootstrap themes on top of the standard Bootstrap offerings like Primary, Secondary, Success, and Danger.
Consider the following example where you add an orange theme and a purple key.
$theme-colors: (
"orange": #ffa500,
"purple": #800080
);
@import "../node_modules/bootstrap/scss/bootstrap";
Now, compiling the SASS will result in a complete Bootstrap stylesheet with these two extra themes included. You can then use them, just as you would any of the standard offerings. The styles are automatically generated with the appropriate text color and hover effects.
<button class="btn btn-orange">Orange</button>
<button class="btn btn-purple">Purple</button>
The Bottom Line
In this tutorial, you learned how Statiq can be used to output documents from files outside the input folder. You can use this technique to include .js and .css managed by npm or other package managers. You also learned an easy technique for compiling Bootstrap from SASS by importing the desired .scss into a new SASS css file. This method allows you to easily customize styling for your project.
Don't stop learning!
There is so much to discover about C#. That's why I am making my favorite tips and tricks available for free. Enter your email address below to become a better .NET developer.
Did you know?
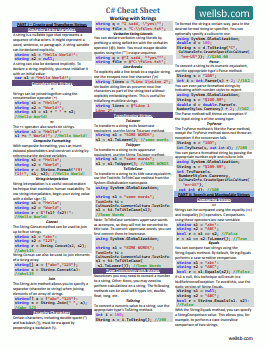
Our beautiful, multi-column C# reference guides contain more than 150 tips and examples to make it even easier to write better code.
Get your cheat sheets